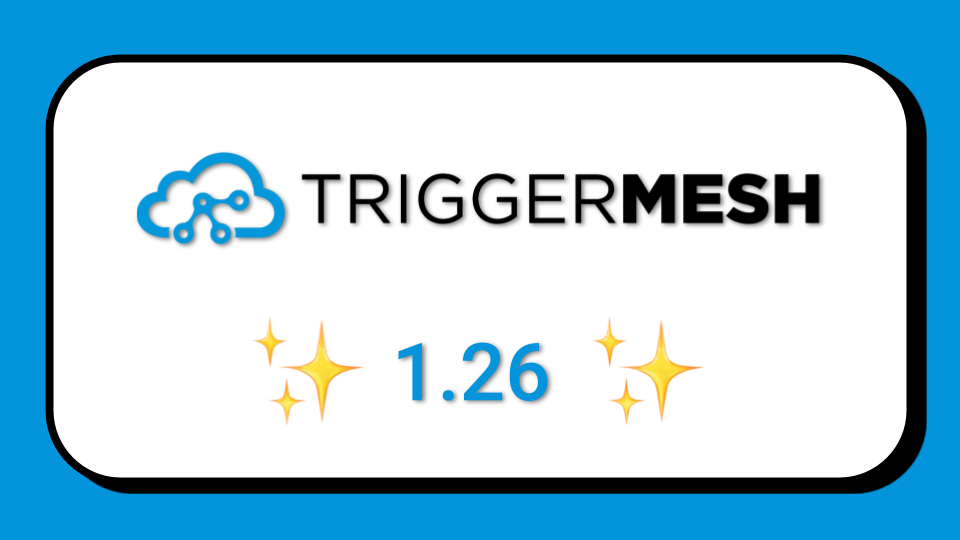
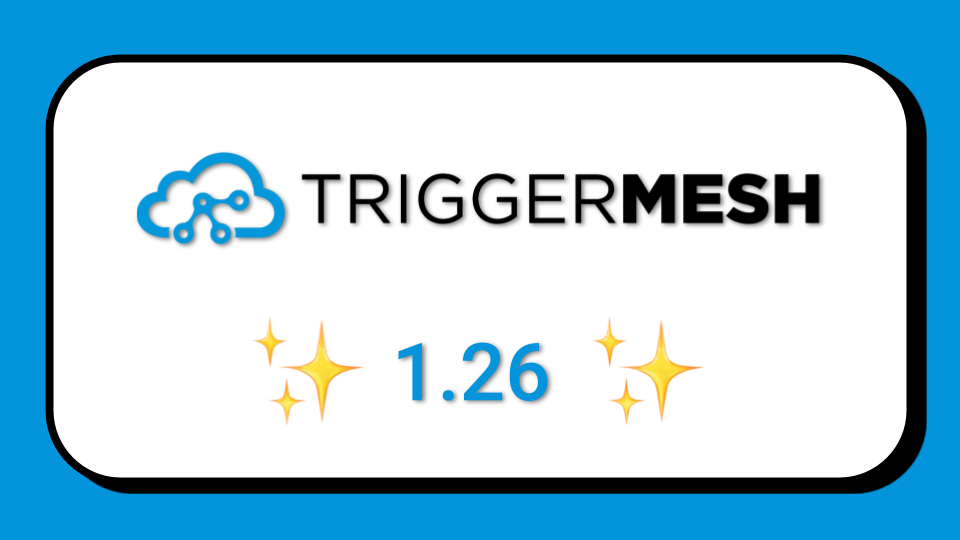
The Kubernetes client SDKs let you perform the same actions as kubectl, but from your preferred programming language. This provides a more programmatic approach that can be suitable for creating integrations on the fly, for example on behalf of end-users of your application.
Let’s look at how to programmatically create some TriggerMesh integrations with a Kubernetes SDK for Node.js. We’ll go through the following:
All the example code is provided on GitHub. At the end of the tutorial, your Kubernetes cluster should be running the configuration shown in the diagram below:
TriggerMesh is an easy way to connect systems together through events, and lets you route and transform events in a unified way, no matter what source they originate from.
TriggerMesh supports two main user interfaces as of today: the command line interface called tmctl, and the Kubernetes API objects. But if you want to programmatically create TriggerMesh integrations from your preferred programming language, you might be looking for a REST API or similar that we don’t support (let us know if this is something you need).
But there are programmatic ways to interact with the Kubernetes API other than the commonly used kubectl CLI. Kubernetes provides a number of supported client SDKs, and there are many community-supported alternatives as well. Lets see how we can use these to achieve programmatic configuration of TriggerMesh resources.
In this tutorial we are going to programmatically create an HTTP Poller event source, that works by polling an external HTTP service and turning the responses into events that are pushed to the Broker.
Prerequisites: TriggerMesh is installed on a Kubernetes cluster. Please refer to the dedicated guides for either YAML or Helm installation.
We’re going to start by manually creating some TriggerMesh components on the Kubernetes cluster. These steps would typically be done by a DevOps engineer. This will establish the basis for later being able to easily instantiate the integration “templates” directly from Node.js code.
First, make sure you’ve installed TriggerMesh on the cluster. Then we’ll proceed to create a namespace for this tutorial:
Create a Broker, a Target that consumes events and displays them (we’ll use this to make sure our event source works), and a Trigger that routes events from Broker to Target:
Starting by checking out the tutorial from GitHub:
And move into the project folder:
Install the dependencies:
We’ll use GoDaddy's Kubernetes client for Node.js. Any other Kubernetes library for Node.js that has a dynamic client or supports CRDs can be used. Run the following command to install this package:
Let’s go through the main.js file to see how the project works.
In the first two lines, we’re adding the objects required from the Kubernetes client package:
Lines 4-7, we’re using the TriggerMesh custom resource definition (CRD) for the HTTP Poller event source as a schema to help us create the Kubernetes objects from Javascript code.
For simplicity we’ve provided them as part of the GitHub project (see CRD for HTTP Poller source and resource instance for the HTTP Poller). You can modify these, but keep in mind that the Trigger created will only let event types demo.type1 through.
First we load the schema into the application by loading the CRD. Then we load an instance of the object (i.e. a custom resource) into a variable.
Line 10 we’re assigning the Kubernetes namespace to the NAMESPACE environment variable.
Now for the main function.
Lines 13 to 27 we set up a Kubernetes client using the expected locations on your computer. When running the code inside the cluster the environment variable NODE_ENV=production should be set.
The kubeclient object can now be used to connect to the cluster, but it knows nothing about TriggerMesh components. We need to inform it about the CRD schemas for the objects we want to manage, which we do on line 34:
Lines 38 and 39 we use the kubeclient to call the CRD API (identified by its group and version), at the namespace where we want to create the HTTP Poller event source:
Run the application pointing to the namespace where the RedisBroker is running.
If you are running the application from a Kubernetes Pod, make sure to also set NODE_ENV=production.
After issuing the command a new HTTP Poller event source should be ready to capture events.
Make sure everything is running as expected:
And now to check that the events from the HTTP Poller source are making it into the cluster, we'll check the output logs of the event display service, which should be giving you weather updates:
Here are some ideas of next steps you could take to go beyond this tutorial:
- Playing with the parameters of the HTTP Poller source
- Reading the CRD from the live cluster instead of from a local file
- Adding operations in Node.js to update and delete an existing HTTP Poller source
- Manage other K8s objects from the Node.js code, such as the Redis Broker or Trigger.
The easiest way to clean up would be:
But we would suggest extending the code to remove it programmatically. It is as simple as slightly modifying one line at the provided code!
Let us know what you think on Slack or GitHub, we’d love to hear from you.