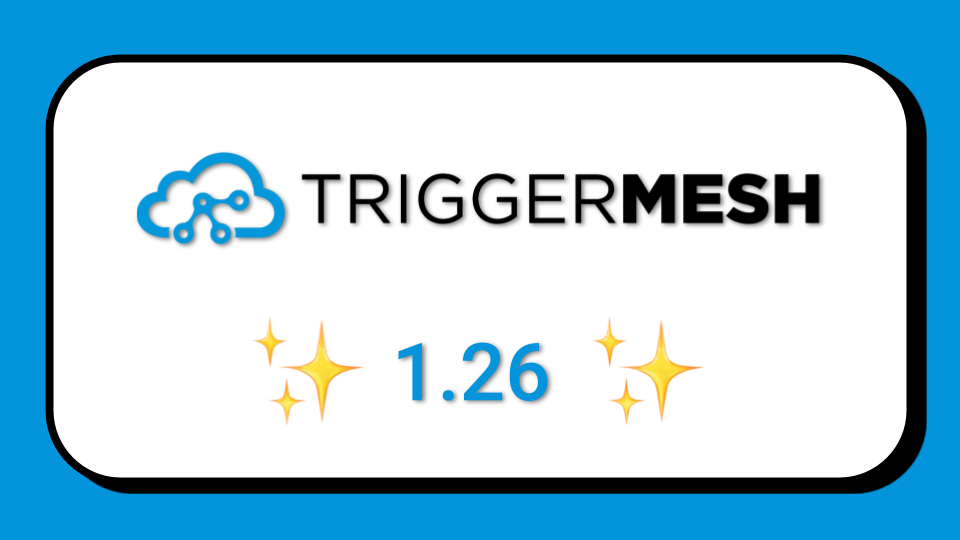
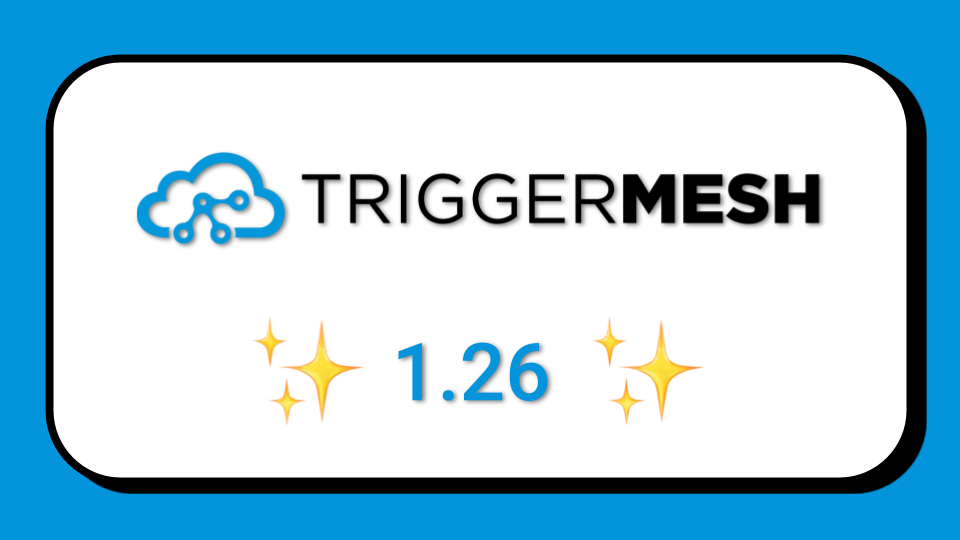
In oder to build event driven applications you need an eventing substrate to connect your event producers and consumers. Traditionally this can be done with Pub/Sub systems common to the enterprise stack like Kafka, Redis, RabbitMQ or other Cloud based solutions like AWS Kinesis. To connect event producers and consumers globally there are also solutions like Synadia NGS or Solace which provide a global secure and scalable network to link all your devices together.
In this blog post we will be looking at an emerging technology a little off the beaten path of the enterprise IT stack called NKN. Specifically, we will be looking at how to leverage the messaging interface that NKN provides to send and receive data using the NKN Golang SDK and therefore ultimately build event-driven applications with Triggermesh sources, targets and transformations.
Before we begin, lets have a small primer on what NKN is.
The official NKN website describes it as: "... a new kind of peer to peer network connectivity protocol and ecosystem powered by a novel public blockchain. It uses economic incentives to motivate Internet users to share network connections and utilize unused bandwidth."
The platform boasts a ton of features, including:
Let's start using NKN step by step, starting from scratch with a consumer in Go.
1. Create a new Go project.
2. Import the NKN SDK.
This can be accomplished by adding the following to your main.go file:
3. Create a new function called start . This function will be called when the program is started and will handle all of the logic in our example program.
4. Begin populating the start function by creating a new account.
5. Create a new client.
NKN does something interesting here. You can create a new client with the use of either nkn.NewClient() or nkn.NewMulticlient().
The nkn.NewClinet() method creates a single standard client, as one might expect. However, the nkn.NewMulticlient() method creates a client that can connect send/receive packets concurrently. This will greatly increase reliability and reduce latency at the cost of more bandwidth usage (proportional to the number of clients).
For this example, we will be using the nkn.NewMulticlient() method.
note the "" parameter in the NewMultiClient() method. This is called an identifier and is used to distinguish different clients sharing the same key pair. Since we will be using two distinct clients, we do not need to include this in the call.
1. Log the client address we just created.
2. Create a loop to listen for incoming messages.
3. Call the start function from the main function.
Your nknconsumer.go file should look something like this:
Run the program and retrieve your client address.
The following shows a sample client address returned at the terminal:
Now that we have a consumer set up and have its address, we can create a producer and start sending messages!
1. Create a new Go project.
2. Import the NKN SDK.
This can be accomplished by adding the following to your main.go file:
3. Create a new function called start. This function will be called when the program is started and will handle all of the logic in our example program.
4. Begin populating the start function by creating a new variable called ca that will pull from an environment variable "CONSUMER_ADDRESS". This will enable us to change the consumer address in the future, without modifying the code.
5. Create a new account.
6. Create a new NKN client from the account and start listening for connections.
7. Send a message to the client.
8. Include some logic to handle the reply
Your nknconsumer.go file should look something like this:
Now that we have a consumer and a producer set up, we can start to send some messages.
1. Launch the nknconsumer.go program and note down the consumer address.
go run .
You will see a new consumer address printed out:
2. Open a new terminal and navigate to the nknproducer directory.
3. Export the consumer address to an environment variable.
4. Launch the nknproducer.go program.
Results will be printed out at both of the consumer and producer terminals and will resemble the following
nknproducer.go
nknconsumer.go
NKN is a "New Kind of Network" built on a public Blockchain technology, it offers a scalable, secure, low latency peer to peer network with a simple pub/sub programming interface that can be used to build event driven applications.
While tied to a cryptocurrency based economy it can be used by TriggerMesh sources, targets, transformations to build highly distributed event-driven applications for the enterprise and provides an alternative to more main stream pub/sub global substrates.